Bootstrap Your Coding Journey: How to Set Up ReactJS
Ready to start your journey with ReactJS? Our in-depth step-by-step guide has everything you need to know to set up ReactJS. Dive in and start coding!
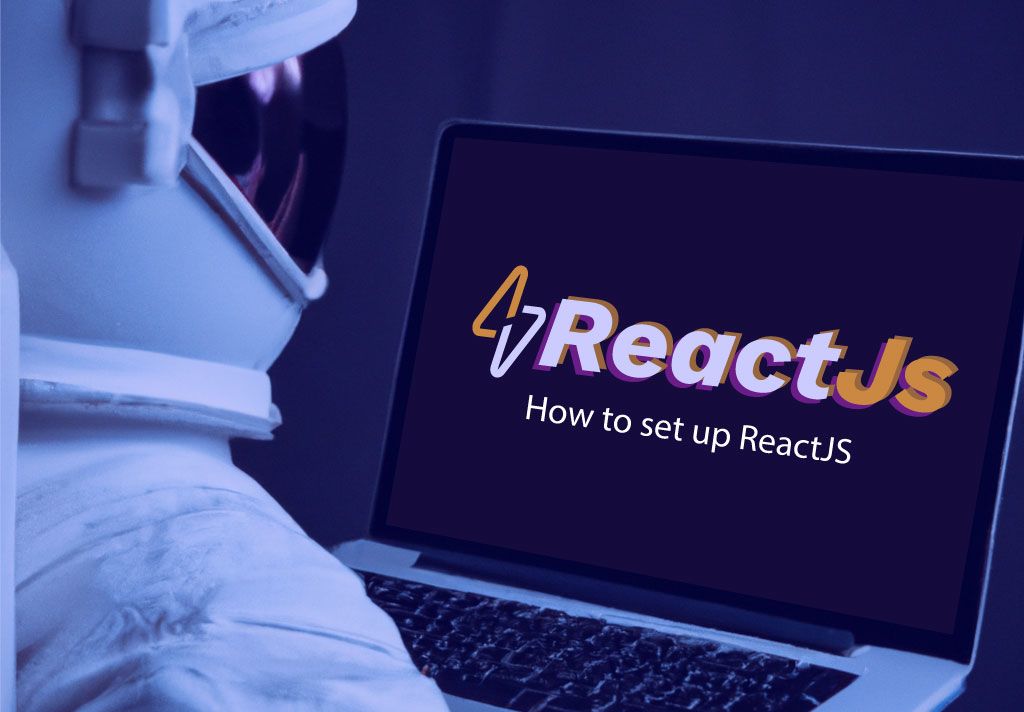
So you’re intrigued by ReactJS and can't wait to dip your toes in? Before you dive in, here's everything you need to know about setting up ReactJS!
Welcome, coding enthusiasts! The world of ReactJS is bustling with potential, and this guide is just what you need to kickstart your journey. If you’re ready to embark on a coding adventure, stick around as we map out a clear path for setting up ReactJS, your golden ticket to crafting stellar web applications.
Prerequisites for Setting Up ReactJS
Buckle Up for the Ride: Dusting Off the JavaScript Blues and Gearing Up!
You know that exciting feeling of embarking on an epic road trip? That's how we feel right now! Before we hit the gas pedal and shoot down the highway of ReactJS setup, there are a few things we need to check off our list. Remember, preparation is key to a smooth journey!
JavaScript Basics
Putting the "JS" in ReactJS: Why the JavaScript Basics Matter
While you don't need to be a JavaScript prodigy to use ReactJS, a clear understanding of the basics will propel your journey. Brush up your knowledge of variables, operators, data types, functions, and loops. Transition smoothly into advanced concepts like ES6 features, including let, const, arrow functions, template literals, and spread operators. Trust us, it'll be like the wind beneath your wings!
System Requirements and Software
Prepping Your Toolbox: System Requirements and Vital Software
Next up is setting up the technical bits necessary for a hassle-free experience with ReactJS:
- Node.js: The foundational stone for your ReactJS house. Make sure you have Node 10.16.0 or later version installed on your system.
- npm or Yarn: These are your package managers, responsible for handling all your project's dependencies. npm gets automatically installed when you install Node.js. If you’re more of a Yarn person, you'll need to install it separately.
- Text Editor: Visual Studio Code, Sublime Text, Atom - pick your weapon of choice! These text editors will be where you write your beautiful ReactJS code.
With the right foundation laid out, it's time to roll up our sleeves and start building!
Step-by-Step Guide to Setting Up ReactJS
The Lego Build: Assembling ReactJS, One Piece at a Time!
Setting up ReactJS is akin to assembling a Lego masterpiece. Follow these incremental steps and voila! You'll have a robust ReactJS set up in no time.
Step 1: Installing Node.js and npm/yarn
Heading 3: Laying the Foundation: Installing Node.js and npm/yarn
First up, let's install Node.js and npm. Verified that you've got them installed? Great, let's move to the next phase!
Installing Node.js
Before installing Node.js, make sure you don't already have it installed on your system by running the following command:
node -v
If Node.js isn't installed, head over to the Node.js official website and download the latest LTS (Long-Term Support) version compatible with your operating system. Follow the installation instructions provided.
Once the installation is complete, open your terminal or command prompt and verify it by running:
node -v
You should see the version number of the installed Node.js displayed.
Installing npm
When you install Node.js, npm is automatically installed as well. You can verify the installation with the following command:
npm -v
This will display the version number of npm.
Installing Yarn (Optional)
Yarn is an alternative package manager to npm. It's optional but can provide faster package installation and improved security. If you'd like to use Yarn, follow these installation steps:
- Open your terminal or command prompt.
- Run the following command:
npm install -g yarn
- Verify the installation by running:
yarn -v
This will display the version number of Yarn.
Now that Node.js and npm/yarn are installed successfully, proceed to the next steps to set up ReactJS.
Step 2: Installing create-react-app
Building the Framework: Installing create-react-app
Next, we'll install create-react-app, a toolset built by Facebook that helps set up a new ReactJS project. Think of it as your coding Swiss knife! It's extremely helpful and saves you from the grunt work by setting up a development environment and optimising your app for production.
Let's get right to it then! Setting up ReactJS just got a whole lot easier thanks to Facebook's create-react-app
toolset. So, let's get this installed.
Installing create-react-app Globally
Open your terminal and type in the following command:
npm install -g create-react-app
Alternatively, if you're using Yarn, use:
yarn global add create-react-app
This command installs create-react-app
globally on your computer, allowing you to create new projects from anywhere on your system.
Verifying the Installation
Check that create-react-app
is installed correctly and ready to be used by running:
create-react-app --version
You should see the version number of create-react-app
displayed.
And that's it! You've now installed create-react-app
, making it a breeze to initialise new ReactJS projects. You're all set and ready to create your first ReactJS application.
Step 3: Creating a New ReactJS Application
Heading 3: Breathing Life into Your Project: Creating a New ReactJS Application
With the environment set, it's time to bring our project to life. We'll create a new application using the command line tool we just set up. Here's where you'll see the power of create-react-app!
Creating a new ReactJS application involves a simple command-line command: npx create-react-app [app-name]
. The npx
package, bundled with npm
version 5.2 and later, facilitates creating a new React application.
Here's the detailed procedure:
- Open your terminal, then navigate to the directory where you want your application to reside.
- Run the following command to create a new React application:
npx create-react-app my-app
Note: Replace "my-app" with the distinct name of your application. React uses the name you provide to create a directory that corresponds to the ".js" file generated.
- Once the process finishes, navigate into your newly created app directory:
cd my-app
- Start your application:
npm start
After running this command, your React application should open automatically in your default web browser. If the application doesn't open automatically, navigate to http://localhost:3000 in your browser.
Congratulations! You've created your first ReactJS application using create-react-app
.
So, take some time to explore the generated files and familiarize yourself with the resulting project structure!
Step 4: Understanding the Project Structure
A Tour Around the House: Understanding the Project Structure
Now that our app has materialized, let's take a tour around our project structure. This will help you understand where to find what, setting the stage for writing and managing your code efficiently.
After creating your new ReactJS application using create-react-app
, it's essential to understand the file and folder structure that got generated. Let's check out what each file and folder does:
- node_modules: This folder contains all of the packages of code that your project depends on (npm packages) are automatically installed.
- public: This folder contains static files and assets that aren't processed by Webpack.
- index.html: The main HTML file. Your React app renders inside a div with an id of 'root' in this file. You can add public assets in this file.
- favicon.ico: The icon you see in the browser tab.
- manifest.json: It's used for configuring how your app appears and how it's launched when your app is installed on a user's mobile or desktop device.
- src: This folder contains all of your React code.
- App.js: This file is the main component of your application. This is where you'll implement the bulk of your React code.
- index.js: The JavaScript entry point. It renders the
<App />
component to our root div inindex.html
. - serviceWorker.js: This is an automatically included script that makes your app work offline and load faster. It's also known as a 'Progressive Web App'.
- package.json: This file holds various metadata relevant to the project, including its name, version and an array of dependencies packages essential to running your project. If you want to add more dependencies, you can do it here.
- README.md: Documentation on how to run and build the project.
- .gitignore: This text file tells Git which files it should not track or maintain.
With a good understanding of the project structure, you can now write code more efficiently and navigate smoothly around your ReactJS application. Ready for the next step – running the application?
Step 5: Running the React Application
The Drumroll Moment: Running the React Application
Finally, the moment we've been waiting for! Let's fire up our application and see it run. Wait till you see how easy this is!
Running your React application locally is a simple process with create-react-app
. Here's how to do it:
- Open your terminal or command prompt.
- Navigate to your project directory using the
cd
command. For example, if you named your application "my-app", you will write:
cd my-app
- Once you're in the appropriate directory, start your React app using npm start or yarn start depending on the package manager you're using.
With npm, run the following:
npm start
With yarn, run the following:
yarn start
- After running the command, your application should start and automatically open in your default web browser. If it doesn't open automatically, you can manually open your browser and navigate to
"localhost:3000"
.
You should see the basic React app screen. From here you have everything set up and ready for you to start building your ReactJS Application!
Important to note: While npm start
or yarn start
is running, any changes you make to your files will automatically refresh the page in your browser so you can see the changes in real-time.
Finally, to stop the server from running, you can hit "CTRL + C" in your terminal.
Now, you're up and running with your own ReactJS application, ready to code away and explore the limitless world of ReactJS!
Troubleshooting Common Issues
The Guide for Coding Pitfalls: Troubleshooting Common Issues
Even though setting up ReactJS is primarily a smooth process, you might face some challenges along the way. Don't worry, with this guide, you're equipped to get past any roadblocks you may encounter.
Occasionally, you might encounter issues while working with ReactJS applications. Here are some common problems and their respective solutions:
1. The app doesn't run or start on the desired port
By default, your React app runs on port 3000
. If that port is unavailable, you might encounter issues starting your app. To resolve this, you can change the port number before running npm start
or yarn start
:
For npm:
export PORT=4000 && npm start
For yarn:
PORT=4000 yarn start
Replace 4000
with the desired port number.
2. The app doesn't refresh automatically after changes in the file
If your app doesn't refresh automatically after your code updates, there could be an issue with the watch feature. To fix this, try deleting the node_modules
folder and reinstalling dependencies using npm install
or yarn install
. After successfully reinstalling, start the app again with npm start
or yarn start
.
3. The package installation fails or throws an error
Occasionally, package installations might fail or return errors. To troubleshoot this:
- Verify your Node.js and npm/yarn are up-to-date. You can update Node.js by visiting the Node.js official website and following the installation instructions. To update npm, type
npm install -g npm@latest
. For Yarn,npm install -g yarn
. - Clear the npm cache with
npm cache clean --force
. - Remove the
node_modules
folder and reinstall dependencies using thenpm install
oryarn install
command. - Try using a stable internet connection and verify there is no proxy or VPN interference during the installation process.
4. The create-react-app
command isn't recognized
If the create-react-app
command is not recognized after the global installation, make sure your $PATH
environment variable includes the global npm modules directory.
For npm:
export PATH=$PATH:$(npm -g bin)
For yarn, ensure it is added to the path:
export PATH="$(yarn global bin):$PATH"
Add the appropriate line to your system's shell configuration file (e.g., .bashrc
, .zshrc
, etc.) to persist the changes.
Armed with these troubleshooting strategies, you'll be able to overcome many common issues while working on your ReactJS projects.
Installation Problems
Smoothing Out the Bumps: Addressing Installation Problems
Some developers encounter installation issues related to incorrect versions or problems with npm/yarn. We’ll cover common installation hitches and how to overcome them.
While setting up your ReactJS development environment, you might encounter some installation-related problems. Here are a few common issues and their solutions:
1. Failure to install create-react-app
globally
If you're having trouble installing create-react-app
, try clearing your npm cache and reinstalling the create-react-app
with the following commands:
npm cache clean --forcenpm install -g create-react-app
2. Errors during React project creation
If you run into issues when executing the create-react-app
command to create your project, ensure you have a stable internet connection and that there is no proxy or VPN interference. Also, double-check your Node.js and npm installations are up-to-date.
3. npx
isn't recognized or throws an error
If you face issues using npx
, you may need to update your Node.js and npm installations. Visit the Node.js official website to download and update your Node.js version. To update npm, type npm install -g npm@latest
.
4. Permissions errors during installation
In certain cases, you might deal with permission errors during the installation of global npm packages. To resolve this issue, you can either:
- Use your system's package manager to install Node.js, following the instructions in the official Node.js documentation.
- Configure npm to use a different location to install global packages without using sudo. Follow the directions in the official npm documentation.
5. Global installations don't work or are unrecognized
Make sure your $PATH
environment variable includes the global npm modules directory. Add the following line to your system's shell configuration file (e.g., .bashrc
, .zshrc
, etc.):
For npm:
export PATH=$PATH:$(npm -g bin)
For yarn:
export PATH="$(yarn global bin):$PATH"
By addressing these installation problems, you'll be one step closer to successfully setting up and building your ReactJS applications.
Command Errors
Mastering the Command Line: Solving Command Errors
Errors with command lines can put a dent in your progress. Together, we’ll stroll through some common command errors and their solutions.
Remember, no journey is completely obstacle-free. It's all part of the learning process. With this troubleshooting guide by your side, you're all set to tackle these bumps on your way!
While working with ReactJS applications, you might encounter a few command-related issues. Here are some solutions to typical problems:
1. npm start
or yarn start
doesn't work
If the npm start
or yarn start
command fails to run your application, try the following steps:
- Check your
package.json
for thestart
script. It should look like this:
"scripts": { "start": "react-scripts start"}
- Delete
node_modules
andpackage-lock.json
oryarn.lock
, then reinstall your dependencies usingnpm install
oryarn
. - Ensure you have a
public/index.html
and asrc/index.js
file in your project as these are required for the development server to start.
2. create-react-app
is not recognized
If you installed it globally and it is not recognized, make sure your global modules directory is included in your PATH environment variable. Update the PATH with the following commands:
export PATH=$PATH:$(npm -g bin) # For npmexport PATH="$(yarn global bin):$PATH" # For yarn
3. npm run build
errors
If you encounter errors when trying to build your application using npm run build
:
- Ensure the
scripts
section in yourpackage.json
has abuild
script:
"scripts": { "build": "react-scripts build"}
- Delete
node_modules
andpackage-lock.json
oryarn.lock
, then reinstall your dependencies usingnpm install
oryarn
. - Check for any compilation errors in your source code.
Please note that specific errors may require specific solutions, so the error message will often provide the best indication of what needs to be fixed. If an error message isn't clear, it might be helpful to copy and paste it into a web search to learn more about what could be going wrong.
Up Next: Exploring More of ReactJS
The Uncharted Expedition: Exploring More of ReactJS
Congratulations, code ninja! You've set up ReactJS and are now at the looking glass entering a whole new world of possibilities. The setup was your first step - the gateway to exploring more of React. Remember, courage is taking that step into the unknown. Stay tuned as we venture deeper into the realm of ReactJS - exploring components, props, state, and so much more.
Once you've got the basics of creating and running a ReactJS application, it's a good idea to delve deeper into ReactJS concepts and techniques. Here are a few things to explore:
1. React Components
Learn more about creating and using functional and class components, as well as their lifecycle methods, state, and props.
2. Styling in React
Explore different ways of styling your application, including CSS modules, inline styling, and Styled Components.
3. React Router
Study how to manage routing in your single-page React application by using React Router to navigate between components.
4. State Management
Look into advanced state management solutions such as Redux, MobX, and the React Context API to manage complex application state efficiently.
5. React Hooks
Dive into React Hooks like useState
, useEffect
, useReducer
, and custom hooks to manage state and side effects more easily in functional components.
6. Server-side Rendering
Discover how to optimize your React application using server-side rendering with frameworks like Next.js.
7. Performance Optimization
Learn strategies for improving the performance of your React app, such as lazy loading, code splitting, and caching.
8. Testing
Understand how to write tests for your React components using React Testing Library and Jest.
9. Deploying React Apps
Familiarize yourself with the process of deploying your React application to production using various hosting platforms like Netlify, Vercel, or AWS.
As you explore the world of ReactJS, you'll continue to learn, improve, and enhance your skills, empowering you to build a wide range of applications efficiently and effectively.
Conclusion
The End of the Beginning: You've Got ReactJS Set Up!
Setting up ReactJS may have felt like a monumental task before you started. However, now that you're at the other end, you realize it wasn't so daunting. Indeed, the journey of a thousand miles begins with a single step!
As you venture further down the path of web development with React, remember - every great coder was once a beginner. So, head held high, take one step after another and keep coding! The world is yours to create.